第一步、將 FeederAPI.cs 靜態類別加入到方案總管參考專案。
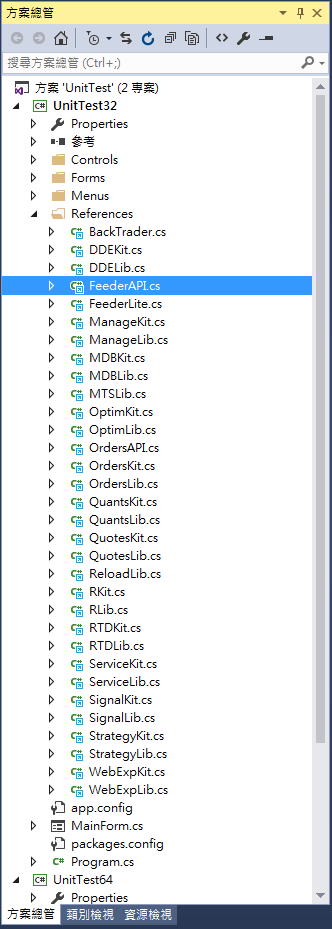
第二步、設計使用者介面,並依建置類型在前置處理器定義 x86 或 x64 指定使用 dll 名稱。
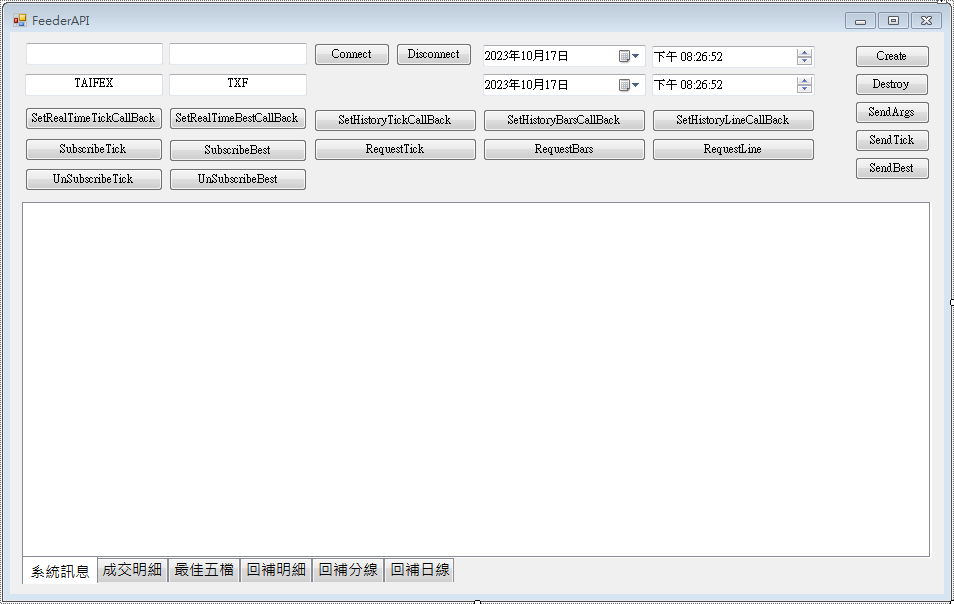
class FeederAPI
建置類型 | x86 | x64 |
元件名稱 | FeederAPI-32.dll | FeederAPI-64.dll |
Methods
int Connect(string host, int port) |
功能 : 建立行情伺服器連線。 參數 : ● host – 伺服器位址 ● port – 伺服器埠口 回傳 : 成功為 0,失敗回傳錯誤碼。 |
int Disconnect() |
功能 : 中斷行情伺服器連線。 回傳 : 成功為 0,失敗回傳錯誤碼。 |
int SendTick(string exch, string code, int date, int time, double price, int volume, double bidprice, int bidvolume, double askprice, int askvolume, int totalvolume) |
功能 : 發送逐筆明細到行情伺服器。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● price – 成交價 ● tick – 成交單量 ● bidprice – 委買價 ● bidvolume – 委買量 ● askprice – 委賣價 ● askvolume – 委賣量 ● totalvolume – 累積成交量,需越來越大才有效 回傳 : 小於 0 為錯誤碼,成功則回傳待發送筆數。 |
int SendBest(string exch, string code, int date, int time, double bidprice1, int bidvolume1, double bidprice2, int bidvolume2, double bidprice3, int bidvolume3, double bidprice4, int bidvolume4, double bidprice5, int bidvolume5, double askprice1, int askvolume1, double askprice2, int askvolume2, double askprice3, int askvolume3, double askprice4, int askvolume4, double askprice5, int askvolume5) |
功能 : 發送最佳五檔到行情伺服器。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● bidprice1..5 – 委買價一至五檔 ● bidvolume1..5 – 委買量一至五檔 ● askprice1..5 – 委賣價一至五檔 ● askvolume1..5 – 委賣量一至五檔 回傳 : 小於 0 為錯誤碼,成功則回傳待發送筆數。 |
int SubscribeTick(string exch, string code) |
功能 : 新增訂閱逐筆行情。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 回傳 : 小於 0 為錯誤碼,成功則回傳訂閱筆數。 |
int UnSubscribeTick(string exch, string code) |
功能 : 解除訂閱逐筆行情。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 回傳 : 小於 0 為錯誤碼,成功則回傳訂閱筆數。 |
int SubscribeBest(string exch, string code) |
功能 : 新增訂閱最佳五檔。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 回傳 : 小於 0 為錯誤碼,成功則回傳訂閱筆數。 |
int UnSubscribeBest(string exch, string code) |
功能 : 解除訂閱最佳五檔。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 回傳 : 小於 0 為錯誤碼,成功則回傳訂閱筆數。 |
int RequestTick(string exch, string code, int date, int begin_time, int end_time) |
功能 : 回補商品逐筆明細。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期 ● begin_time – 開始時間 ● end_time – 結束時間 回傳 : 小於 0 為錯誤碼,成功則回傳待處理筆數。 |
int RequestBars(string exch, string code, int date, int begin_time, int end_time) |
功能 : 回補商品分線。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期 ● begin_time – 開始時間 ● end_time – 結束時間 回傳 : 小於 0 為錯誤碼,成功則回傳待處理筆數。 |
int RequestLine(string exch, string code, int begin_date, int end_date) |
功能 : 回補商品日線。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● begin_date – 開始日期 ● end_date – 結束日期 回傳 : 小於 0 為錯誤碼,成功則回傳待處理筆數。 |
void SetRealTimeTickCallBack(RealTimeTickCallBack callback) |
功能 : 設定逐筆行情回呼函式。 參數 : ● callback – 委派定義:RealTimeTickCallBack |
void SetRealTimeBestCallBack(RealTimeBestCallBack callback) |
功能 : 設定最佳五檔回呼函式。 參數 : ● callback – 委派定義:RealTimeBestCallBack |
void SetHistoryTickCallBack(HistoryTickCallBack callback) |
功能 : 設定回補商品逐筆回呼函式。 參數 : ● callback – 委派定義:HistoryTickCallBack |
void SetHistoryBarsCallBack(HistoryBarsCallBack callback) |
功能 : 設定回補商品分線回呼函式。 參數 : ● callback – 委派定義:HistoryBarsCallBack |
void SetHistoryLineCallBack(HistoryLineCallBack callback) |
功能 : 設定回補商品日線回呼函式。 參數 : ● callback – 委派定義:HistoryLineCallBack |
Delegate
delegate void RealTimeTickCallBack(string exch, string code, int date, int time, double price, int volume, double bidprice, int bidvolume, double askprice, int askvolume, int totalvolume) |
功能 : 接收逐筆明細函式。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● price – 成交價 ● tick – 成交單量 ● bidprice – 委買價 ● bidvolume – 委買量 ● askprice – 委賣價 ● askvolume – 委賣量 ● totalvolume – 成交量 |
delegate void RealTimeBestCallBack(string exch, string code, int date, int time, double bidprice1, int bidvolume1, double bidprice2, int bidvolume2, double bidprice3, int bidvolume3, double bidprice4, int bidvolume4, double bidprice5, int bidvolume5, double askprice1, int askvolume1, double askprice2, int askvolume2, double askprice3, int askvolume3, double askprice4, int askvolume4, double askprice5, int askvolume5) |
功能 : 接收最佳五檔函式。 參數 : ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● bidprice1..5 – 委買價一至五檔 ● bidvolume1..5 – 委買量一至五檔 ● askprice1..5 – 委賣價一至五檔 ● askvolume1..5 – 委賣量一至五檔 |
delegate void HistoryTickCallBack(int index, string exch, string code, int date, int time, double price, int ticks, int volume, double bidprice, double askprice) |
功能 : 接收回補逐筆函式。 參數 : ● index – 索引編號從大到小,0 表示已回補完成 ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● price – 成交價 ● tick – 單量 ● volume – 成交量 ● bidprice – 委買價 ● askprice – 委賣價 |
delegate void HistoryBarsCallBack(int index, string exch, string code, int date, int time, double open, double high, double low, double close, int totalticks, int totalvolume) |
功能 : 接收回補分線函式。 參數 : ● index – 索引編號從大到小,0 表示已回補完成 ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● time – 交易時間,格式:HHmmss ● open – 開盤價 ● high – 最高價 ● low – 最低價 ● close – 收盤價 ● totalticks – 成交筆數 ● totalvolume – 總成交量 |
delegate void HistoryLineCallBack(int index, string exch, string code, int date, double open, double high, double low, double close, int totalticks, int totalvolume, int openinterest) |
功能 : 接收回補日線函式。 參數 : ● index – 索引編號從大到小,0 表示已回補完成 ● exch – 交易所代碼 ● code – 商品代碼 ● date – 交易日期,格式:yyyyMMdd ● open – 開盤價 ● high – 最高價 ● low – 最低價 ● close – 收盤價 ● totalticks – 成交筆數 ● totalvolume – 總成交量 ● openinterest – 未平倉量 |
- 測試影片: